SpringBoot 整合 Redis
SpringBoot 整合 Redis
简单介绍
前面我们基本把Redis 的基本知识介绍完了,但是可以看出,全部都是在SSH命令行操作的,作为一个学习Java的程序员,肯定希望使用Java来操作一把。我们这里直接使用SpringDataRedis来玩,不在单独使用Jedis来操作了。
项目搭建
创建项目
创建项目我们使用 SpringBoot的引导器来创建,这里就不多赘述了。如何创建SpringBoot项目可以参考。
https://blog.csdn.net/ooyhao/article/details/82898973
导入依赖
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.10.2</version> </dependency>
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.10.2</version> </dependency>
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.10.2</version> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
|
依赖分为两个部分,第一个部分就是jackson-databind,jackson-core,jackson-annotations
关于jackson的几个,第二个部分就是SpringBoot整合redis的staterspring-boot-starter-data-redis
。
配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.database=0
spring.redis.password=
spring.redis.jedis.pool.max-idle=8
spring.redis.jedis.pool.max-wait=-1
spring.redis.jedis.pool.max-active=8
spring.redis.jedis.pool.min-idle=0
|
配置类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| package com.ouyanghao.springbootredis.config;
import com.fasterxml.jackson.annotation.JsonAutoDetect; import com.fasterxml.jackson.annotation.PropertyAccessor; import com.fasterxml.jackson.databind.ObjectMapper; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.data.redis.connection.RedisConnectionFactory; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.data.redis.core.StringRedisTemplate; import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer; import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration public class RedisConfig {
@Bean @SuppressWarnings("all") public RedisTemplate<String,Object> redisTemplate(RedisConnectionFactory factory){
Jackson2JsonRedisSerializer<Object> jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer<Object>(Object.class);
ObjectMapper objectMapper = new ObjectMapper(); objectMapper.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY); objectMapper.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL); jackson2JsonRedisSerializer.setObjectMapper(objectMapper);
RedisTemplate<String,Object> template = new RedisTemplate<>(); template.setConnectionFactory(factory); template.setKeySerializer(new StringRedisSerializer()); template.setValueSerializer(jackson2JsonRedisSerializer); template.setHashKeySerializer(new StringRedisSerializer()); template.setHashValueSerializer(jackson2JsonRedisSerializer); template.afterPropertiesSet(); return template; }
@Bean public StringRedisTemplate stringRedisTemplate(RedisConnectionFactory factory){ StringRedisTemplate stringRedisTemplate = new StringRedisTemplate(); stringRedisTemplate.setConnectionFactory(factory); return stringRedisTemplate; } }
|
封装组件类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| package com.ouyanghao.springbootredis.test;
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.stereotype.Component;
@Component public class RedisListOps {
@Autowired private RedisTemplate<String,Object> redisTemplate;
public void leftPush(String key, Object value){ redisTemplate.boundListOps(key).leftPush(value); }
public void leftPushAll(String key, Object...value){ redisTemplate.boundListOps(key).leftPushAll(value); }
public Object leftPop(String key){ return redisTemplate.boundListOps(key).leftPop(); } }
|
这个组件类是以操作List列表创建的,就不单独每一种类型都创建一个了。
项目测试
测试代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| package com.ouyanghao.springbootredis;
import com.ouyanghao.springbootredis.test.RedisListOps; import org.junit.jupiter.api.Test; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest class SpringbootRedisApplicationTests {
@Autowired private RedisListOps redisListOps;
@Test void contextLoads() { redisListOps.leftPush("animal","apple"); redisListOps.leftPushAll("animal","orange","banana");
} }
|
测试结果
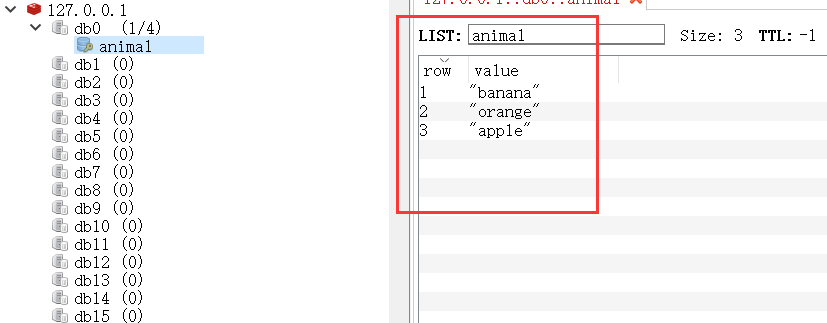
使用SpringBoot整合Redis就先到这里了。