SpringBoot之系统启动任务
什么是系统启动任务呢?其实就是在系统启动的时候需要执行的任务代码,而后面都不会再执行到的,就成为系统启动任务。
监听器
在 Servlet/Jsp 项目中,如果涉及到系统任务,例如在项目启动阶段要做一些数据初始化操作,这些操作有一个共同的特点,只在项目启动时进行,以后都不再执行,这里,容易想到web基础中的三大组件( Servlet、Filter、Listener )之一 Listener ,这种情况下,一般定义一个 ServletContextListener,然后就可以监听到项目启动和销毁,进而做出相应的数据初始化和销毁操作,例如下面这样:
1 2 3 4 5 6 7 8 9 10
| public class IListener implements ServletContextListener { @Override public void contextInitialized(ServletContextEvent sce) { } @Override public void contextDestroyed(ServletContextEvent sce) { } }
|
当然,这是基础 web 项目的解决方案,如果使用了 Spring Boot,那么我们可以使用更为简便的方式。Spring Boot 中针对系统启动任务提供了两种解决方案,分别是 CommandLineRunner 和 ApplicationRunner,分别来看。
CommandLineRunner
使用CommandLineRunner 创建系统启动任务还是很简单的。如下,我们创建两个系统启动任务:
ICommandLineRunner1:
1 2 3 4 5 6 7 8 9
| @Component @Order(99) public class ICommandLineRunner1 implements CommandLineRunner { @Override public void run(String... args) throws Exception { System.out.println("ICommandLineRunner1:"+ Arrays.toString(args)); System.out.println("系统启动时执行ICommandLineRunner1"); } }
|
ICommandLineRunner2:
1 2 3 4 5 6 7 8 9 10
| @Component @Order(98) public class ICommandLineRunner2 implements CommandLineRunner { @Override public void run(String... args) throws Exception { System.out.println("ICommandLineRunner2:"+Arrays.toString(args)); System.out.println("系统启动时执行ICommandLineRunner2"); } }
|
首先,
- 我们通过自定义类实现CommandLineRunner接口并是实现run方法。run方法中定义的就是系统启动时需要执行的代码。
- Order指明其执行的优先级顺序。Order的数值最大,优先级越低。
- args参数,其实就是SpringBoot的main方法传入的参数。
其他的就不再赘述,说一下参数的问题。
参数可以在main方法的代码中指定。
可以在idea开发环境中指定。多个参数换行指定。
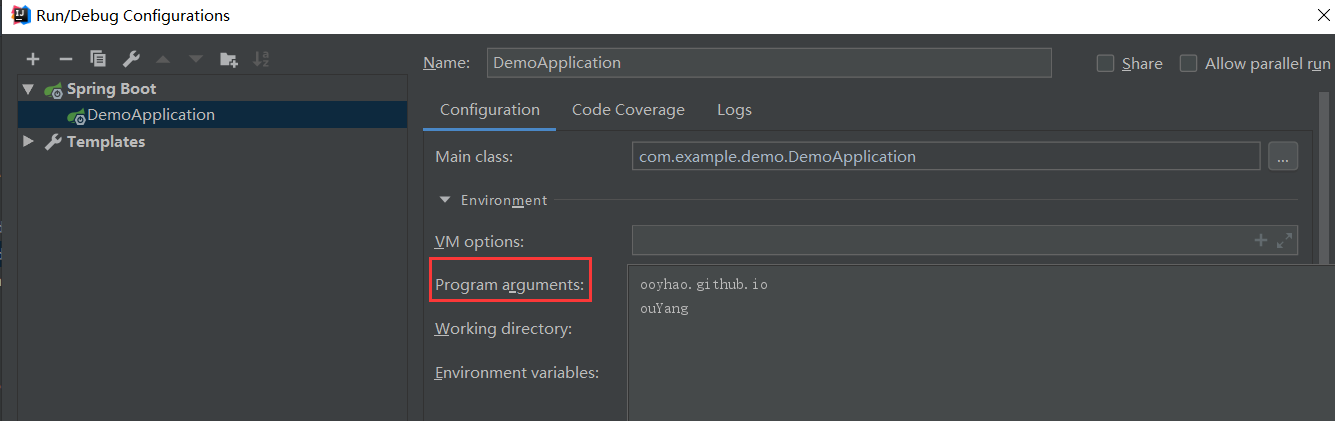
可以在jar包启动的时候执行。多个参数空格指定。(打包 maven package)

ApplicationRunner
ApplicationRunner大致与CommandLineRunner 是一样的,但是在接收参数的时候,有些不同,下面我们实验一下。首先自定义类实现ApplicationRunner 并实现其run方法,同样,run方法就是系统启动任务需要执行的方法。
IApplicationRunner1:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| @Component @Order(99) public class IApplicationRunner1 implements ApplicationRunner { @Override public void run(ApplicationArguments args) throws Exception { String[] sourceArgs = args.getSourceArgs(); System.out.println("sourceArgs:"+Arrays.toString(sourceArgs)); List<String> stringList = args.getNonOptionArgs(); System.out.println("NonOptionArgs:"+stringList); Set<String> optionNames = args.getOptionNames(); System.out.println("========================"); for (String optionName : optionNames){ System.out.println("optionName:" + optionName + ":" + args.getOptionValues(optionName)); } System.out.println("IApplicationRunner1结束"); } }
|
IApplicationRunner2:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| @Component @Order(98) public class IApplicationRunner2 implements ApplicationRunner {
@Override public void run(ApplicationArguments args) throws Exception { String[] sourceArgs = args.getSourceArgs(); System.out.println("sourceArgs:" + Arrays.toString(sourceArgs)); List<String> stringList = args.getNonOptionArgs(); System.out.println("NonOptionArgs:" + stringList); Set<String> optionNames = args.getOptionNames(); System.out.println("========================"); for (String optionName : optionNames) { System.out.println("optionName:" + optionName + ":" + args.getOptionValues(optionName)); } System.out.println("IApplicationRunner2结束"); } }
|
同样,我们利用maven package进行打包,然后看结果,命令如下:
1 2
| java -jar springboot-applicationrunner-0.0.1-SNAPSHOT.jar --name=ooyhao --address=ooyhao.github.io 三国演义 红楼梦
|
其中,--name=ooyhao
和 --address=ooyhao.github.io
就是有key的参数。三国演义
和 红楼梦
就是无key值得参数。
执行结果:
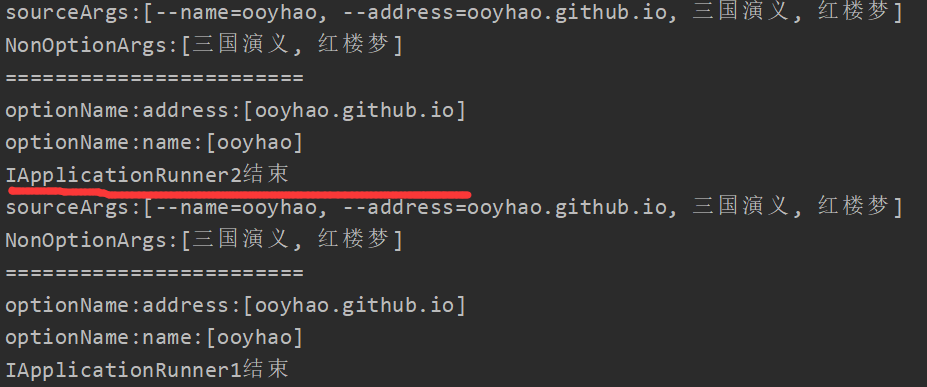
通过结果同样可以看出:
- sourceArgs 表示的是全部参数。
- nonOptionsArgs 表示的是无key的参数。
- optionNames 表示所有key 的name。
拓展可以查看:https://www.javaboy.org/2019/0415/springboot-commandlinerunner.html